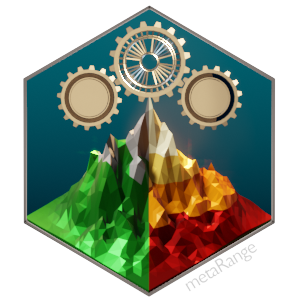
Ricker reproduction model with Allee effects
Source:R/RcppExports.R
ricker_allee_reproduction_model.Rd
An implementation of the Ricker reproduction model with Allee effects based on (Cabral and Schurr, 2010) with variable overcompensation and an extension to handle negative reproduction rates.
Usage
ricker_allee_reproduction_model(
abundance,
reproduction_rate,
carrying_capacity,
allee_threshold,
overcomp_factor = as.numeric(c(1))
)
Arguments
- abundance
<numeric>
vector (or matrix) of abundances.- reproduction_rate
<numeric>
vector (or matrix) of reproduction rates.- carrying_capacity
<numeric>
vector (or matrix) of carrying capacities.- allee_threshold
<numeric>
vector (or matrix) of Allee thresholds.- overcomp_factor
<numeric>
overcompensation factor (default: 1.0). Higher values lead to stronger overcompensation. Can also be a vector or matrix.
Details
Equations:
If \(reproduction\_rate >= 0\) (based on: Cabral and Schurr, 2010): $$N_{t+1} = N_t e^{b r \frac{(K - N_t)(N_t - C)}{(K - C)^2})}$$
If \(reproduction\_rate < 0\): $$N_{t+1} = N_t \cdot e^{r}$$
With:
\(N_t\) = abundance at time t
\(N_{t+1}\) = abundance at time t+1
\(r\) = reproduction rate
\(K\) = carrying capacity
\(C\) = (critical) Allee threshold
\(b\) = overcompensation factor
Note that:
abundance
should generally be greater than 0.reproduction_rate
,carrying_capacity
andallee_threshold
should either all have the same size as the input abundance or all be of length 1.carrying_capacity
should be greater than 0. If it is 0 or less, the abundance will be set to 0.allee_threshold
should be less thancarrying_capacity
. If it is greater than or equal, the abundance will be set to 0.
Important Note:
To optimize performance, the functions modifies the abundance in-place.
This mean the input abundance will be modified (See Examples).
Since the result of this function is usually assigned to the same variable as the input abundance, this is unnoticable in most use cases.
Should you wish to keep the input abundance unchanged, you can rlang::duplicate()
it before passing it to this function.
References
Cabral, J.S. and Schurr, F.M. (2010) Estimating demographic models for the range dynamics of plant species. Global Ecology and Biogeography, 19, 85–97. doi:10.1111/j.1466-8238.2009.00492.x
Examples
ricker_allee_reproduction_model(
abundance = 50,
reproduction_rate = 2,
carrying_capacity = 100,
allee_threshold = -100
)
#> [1] 72.74957
ricker_allee_reproduction_model(
abundance = 50,
reproduction_rate = 2,
carrying_capacity = 100,
allee_threshold = -100,
overcomp_factor = 4
)
#> [1] 224.0845
ricker_allee_reproduction_model(
abundance = matrix(10, 5, 5),
reproduction_rate = 0.25,
carrying_capacity = 100,
allee_threshold = 20
)
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 9.654546 9.654546 9.654546 9.654546 9.654546
#> [2,] 9.654546 9.654546 9.654546 9.654546 9.654546
#> [3,] 9.654546 9.654546 9.654546 9.654546 9.654546
#> [4,] 9.654546 9.654546 9.654546 9.654546 9.654546
#> [5,] 9.654546 9.654546 9.654546 9.654546 9.654546
ricker_allee_reproduction_model(
abundance = matrix(10, 5, 5),
reproduction_rate = matrix(seq(-0.5, 0.5, length.out = 25), 5, 5),
carrying_capacity = matrix(100, 5, 5),
allee_threshold = matrix(20, 5, 5)
)
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 6.065307 7.470175 9.200444 9.825755 9.542067
#> [2,] 6.323367 7.788008 9.591895 9.768350 9.486320
#> [3,] 6.592406 8.119363 10.000000 9.711281 9.430898
#> [4,] 6.872893 8.464817 9.941578 9.654546 9.375801
#> [5,] 7.165313 8.824969 9.883496 9.598141 9.321025
ricker_allee_reproduction_model(
abundance = matrix(10, 5, 5),
reproduction_rate = matrix(1, 5, 5),
carrying_capacity = matrix(100, 5, 5),
allee_threshold = matrix(seq(0, 100, length.out = 25), 5, 5)
)
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 10.941743 8.559290 4.3276907 3.473526e-01 4.799137e-10
#> [2,] 10.588299 7.866279 3.3314695 1.015286e-01 4.102932e-19
#> [3,] 10.180115 7.090663 2.3692776 1.602936e-02 1.082112e-45
#> [4,] 9.710399 6.234417 1.5073508 8.610010e-04 5.705942e-193
#> [5,] 9.172273 5.306779 0.8162661 5.904387e-06 0.000000e+00
ricker_allee_reproduction_model(
abundance = matrix(10, 5, 5),
reproduction_rate = matrix(seq(0, -2, length.out = 25), 5, 5),
carrying_capacity = matrix(100, 5, 5),
allee_threshold = matrix(20, 5, 5)
)
#> [,1] [,2] [,3] [,4] [,5]
#> [1,] 10.000000 6.592406 4.345982 2.865048 1.888756
#> [2,] 9.200444 6.065307 3.998497 2.635971 1.737739
#> [3,] 8.464817 5.580351 3.678794 2.425211 1.598797
#> [4,] 7.788008 5.134171 3.384654 2.231302 1.470965
#> [5,] 7.165313 4.723666 3.114032 2.052897 1.353353
# Note that the input abundance is modified in-place
abu <- 10
res <- ricker_allee_reproduction_model(
abundance = abu,
reproduction_rate = 0.25,
carrying_capacity = 100,
allee_threshold = -100
)
stopifnot(identical(abu, res))